React Anti-Patterns
2024년 01월 05일 출간
- eBook 상품 정보
- 파일 정보 PDF (15.01MB)
- ISBN 9781805123972
- 지원기기 교보eBook App, PC e서재, 리더기, 웹뷰어
-
교보eBook App
듣기(TTS) 가능
TTS 란?텍스트를 음성으로 읽어주는 기술입니다.
- 전자책의 편집 상태에 따라 본문의 흐름과 다르게 텍스트를 읽을 수 있습니다.
- 이미지 형태로 제작된 전자책 (예 : ZIP 파일)은 TTS 기능을 지원하지 않습니다.
PDF 필기가능 (Android, iOS)
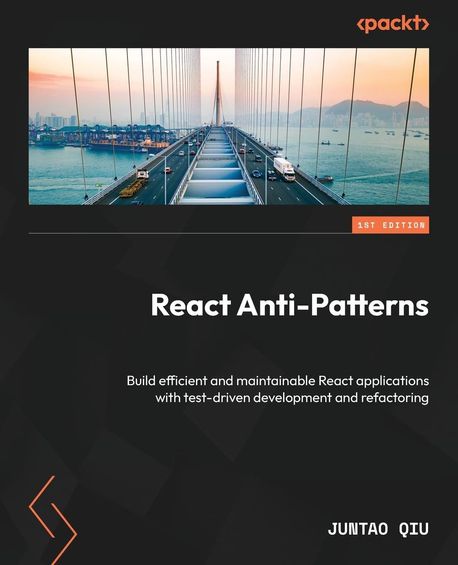
쿠폰적용가 17,100원
10% 할인 | 5%P 적립이 상품은 배송되지 않는 디지털 상품이며,
교보eBook앱이나 웹뷰어에서 바로 이용가능합니다.
카드&결제 혜택
- 5만원 이상 구매 시 추가 2,000P
- 3만원 이상 구매 시, 등급별 2~4% 추가 최대 416P
- 리뷰 작성 시, e교환권 추가 최대 200원
작품소개
이 상품이 속한 분야
▶Book Description
Take your React development skills to the next level by examining common anti-patterns with expert insights and practical solutions, to refine your codebases into sophisticated and scalable creations. Through this easy-to-follow guide, React Anti-Patterns serves as a roadmap to elevating the efficiency and maintainability of your React projects.
You’ll begin by familiarizing yourself with the essential aspects of React before exploring strategies for structuring React applications and creating well-organized, modular, and easy-to-maintain codebases. From identifying and addressing common anti-patterns using refactoring techniques to harnessing the power of test-driven development (TDD), you’ll learn about the tools and techniques necessary to create reliable and robust tests. As you advance, you’ll get to grips with business logic and design patterns that offer solutions to prevalent challenges faced in React development. The book also offers insights into using composition patterns, such as code splitting and multiple entry points, to enhance the flexibility and modularity of your React applications, guiding you through end-to-end project implementation.
By the end of this React book, you’ll be able to overcome common challenges and pitfalls to transform your React projects into elegant, efficient, and maintainable codebases.
▶ What You Will Learn
⦁ Formulate comprehensive testing strategies and leverage testing framework capabilities
⦁ Implement TDD practices to drive the development process and elevate code quality, especially in extensive React projects
⦁ Use design patterns effectively to create scalable and reusable React components
⦁ Apply established software design principles to craft resilient applications within React
⦁ Achieve modularity and loose coupling in React codebases by mastering the separation of concerns
⦁ Ensure clean code by adhering to software design best practices in React development
1. Introducing React Anti-Patterns
2. Understanding React Essentials
3. Organizing Your React Application
4. Designing your React Components
5. Testing in React
6. Exploring Common Refactoring Techniques
7. Introducing Test-Driven Development with React
8. Exploring Data Management in React
9. Applying Design Principles in React
10. Diving Deep into Composition Patterns
11. Introducing Layered Architecture in React
12. Implementing an End-To-End Project
13. Recapping Anti-Pattern Principles
▶ What this book covers
⦁ In Chapter 1, Introducing React Anti-Patterns, you’ll get a closer look at the hurdles of building user interfaces, handling state management, addressing “unhappy paths,” and identifying common anti-patterns in React.
⦁ In Chapter 2, Understanding React Essentials, you will delve into the basics of React covering static components, props, UI breakdown, state management, the rendering process, and common React Hooks to lay a solid foundation for subsequent chapters.
⦁ In Chapter 3, Organizing Your React Application, you will learn about different types of project structures in React, exploring their advantages, drawbacks, and practical applications.
⦁ In Chapter 4, Designing your React Components, you will learn to identify common anti-patterns in React component design and explore fundamental design principles including the Single Responsibility Principle and Don’t Repeat Yourself to improve component structure.
⦁ In Chapter 5, Testing in React, you will learn about the significance of software testing, explore various types of tests such as unit, integration, and end-to-end testing, and get acquainted with popular testing tools including Cypress and Jest, setting a strong foundation for complex testing scenarios in React applications.
⦁ In Chapter 6, Exploring Common Refactoring Techniques, you will learn about the essence of refactoring and delve into various refactoring techniques, such as Rename Variable, Extract Variable, and Replace Loop with Pipeline, to enhance code maintainability and readability.
⦁ In Chapter 7, Introducing Test-Driven Development with React, you’ll learn the core principles of test-driven development through a practical example, while building various features of a pizza store’s menu page in a React application.
⦁ In Chapter 8, Exploring Data Management in React, you’ll delve into the common challenges of state management in React, such as business logic leaks and prop drilling, and explore solutions including employing an Anti-Corruption Layer and utilizing the React context API to enhance code maintainability and user experience.
⦁ In Chapter 9, Applying Design Principles in React, you’ll revisit the Single Responsibility Principle, embrace the Dependency Inversion Principle, and understand the application of Command and Query Responsibility Segregation in React to fortify your knowledge of key design principles to aid you in mastering React.
⦁ In Chapter 10, Diving Deep into Composition Patterns, you’ll delve into composition through higher-order components and custom Hooks, and explore the headless component pattern. You’ll gain an appreciation of composition techniques for creating scalable, maintainable, and user-friendly UIs in React.
⦁ In Chapter 11, Introducing Layered Architecture in React, you’ll explore Layered Architecture, delve into Application Concern Layers, define data models, and learn strategy patterns through a practical example, understanding their significance for large-scale applications.
⦁ In Chapter 12, Implementing an End-To-End Project, you’ll traverse the complete process of developing a weather application, from understanding requirements to implementing features such as City Search and Add To Favourite, while ensuring the code remains maintainable, understandable, and extensible.
⦁ In Chapter 13, Recapping Anti-Pattern Principles, we’ll take a concise look back at common anti-patterns, React design patterns, and fundamental principles, and recap the techniques and practices discussed earlier in the book, providing a succinct refresher before you continue applying these insights to your own projects.
▶ Preface
Building frontend applications is challenging, especially when constructing large ones, and the difficulty escalates without proper guidance. Unfortunately, many React-based applications fall into this scenario due to the library’s UI-centric nature, leaving developers to navigate the other complexities of frontend development on their own. There are numerous other considerations such as asynchronous network requests, accessibility, performance, and state management, to name a few. These factors contribute to the complexity of frontend applications. As the scale of the application grows, maintaining the code becomes an arduous task. Adding new features requires considerably more time than it first appears, and identifying defects (and then fixing them) is equally challenging, if not more so.
However, these challenges are surmountable. We can learn to identify common anti-patterns that cause problems, then employ established patterns, design principles, and practices to address and rectify these issues. History teaches us that solutions derived in one field often find relevance in others, especially when it comes to fundamental design principles such as the Single Responsibility Principle, the Dependency Inversion Principle, and Don’t Repeat Yourself. These principles guided the construction of UNIX systems back in the 1970s and Java Swing applications in the 1990s, and they remain valid today. They will undoubtedly continue to be pertinent for future frameworks and libraries.
This book seeks to delve into these problems and examine how established patterns and practices can mitigate the challenges of building large applications. We’ll see how design principles and design patterns can simplify the design, making the code easier to understand, modify, and maintain in the long run. Through this exploration, readers will gain a deeper understanding of how to navigate the multifaceted world of frontend development with React, ensuring their applications are both robust and maintainable.
작가정보
저자(글) Juntao Qiu
Juntao Qiu is an accomplished software developer with over 15 years of industry experience, dedicated to helping others write better code. With a strong passion for crafting maintainable and high-quality code, he has become a trusted resource in the industry. As an author, Juntao has shared his expertise through influential books such as Maintainable React (2022) and Test-Driven Development with React and TypeScript – Second Edition (2023).
He orchestrates a blend of code snippets, illustrations, and before and after comparisons to unravel intricate concepts, making them accessible to fellow developers. His insightful blog posts at https://juntao.substack.com/ and educational videos on YouTube (https://www.youtube.com/@icodeit.juntao) are a testament to his commitment to sharing knowledge and fostering a deeper understanding of coding practices within the developer community.
이 상품의 총서
Klover리뷰 (0)
- - e교환권은 적립일로부터 180일 동안 사용 가능합니다.
- - 리워드는 5,000원 이상 eBook, 오디오북, 동영상에 한해 다운로드 완료 후 리뷰 작성 시 익일 제공됩니다. (2024년 9월 30일부터 적용)
- - 리워드는 한 상품에 최초 1회만 제공됩니다.
- - sam 이용권 구매 상품 / 선물받은 eBook은 리워드 대상에서 제외됩니다.
- 도서나 타인에 대해 근거 없이 비방을 하거나 타인의 명예를 훼손할 수 있는 리뷰
- 도서와 무관한 내용의 리뷰
- 인신공격이나 욕설, 비속어, 혐오 발언이 개재된 리뷰
- 의성어나 의태어 등 내용의 의미가 없는 리뷰
구매 후 리뷰 작성 시, e교환권 100원 적립
문장수집
- 구매 후 90일 이내에 문장 수집 등록 시 e교환권 100원을 적립해 드립니다.
- e교환권은 적립일로부터 180일 동안 사용 가능합니다.
- 리워드는 5,000원 이상 eBook에 한해 다운로드 완료 후 문장수집 등록 시 제공됩니다. (2024년 9월 30일부터 적용)
- 리워드는 한 상품에 최초 1회만 제공됩니다.
- sam 이용권 구매 상품 / 선물받은 eBook / 오디오북·동영상 상품/주문취소/환불 시 리워드 대상에서 제외됩니다.
구매 후 문장수집 작성 시, e교환권 100원 적립
신규가입 혜택 지급이 완료 되었습니다.
바로 사용 가능한 교보e캐시 1,000원 (유효기간 7일)
지금 바로 교보eBook의 다양한 콘텐츠를 이용해 보세요!
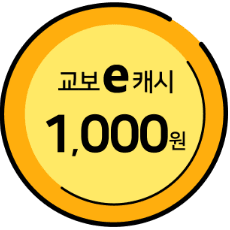
- 구매 후 90일 이내 작성 시, e교환권 100원 (최초1회)
- 리워드 제외 상품 : 마이 > 라이브러리 > Klover리뷰 > 리워드 안내 참고
- 콘텐츠 다운로드 또는 바로보기 완료 후 리뷰 작성 시 익일 제공
가장 와 닿는 하나의 키워드를 선택해주세요.
총 5MB 이하로 jpg,jpeg,png 파일만 업로드 가능합니다.
신고 사유를 선택해주세요.
신고 내용은 이용약관 및 정책에 의해 처리됩니다.
허위 신고일 경우, 신고자의 서비스 활동이 제한될 수
있으니 유의하시어 신중하게 신고해주세요.
이 글을 작성한 작성자의 모든 글은 블라인드 처리 됩니다.
구매 후 90일 이내 작성 시, e교환권 100원 적립
eBook 문장수집은 웹에서 직접 타이핑 가능하나, 모바일 앱에서 도서를 열람하여 문장을 드래그하시면 직접 타이핑 하실 필요 없이 보다 편하게 남길 수 있습니다.
차감하실 sam이용권을 선택하세요.
차감하실 sam이용권을 선택하세요.
선물하실 sam이용권을 선택하세요.
-
보유 권수 / 선물할 권수0권 / 1권
-
받는사람 이름받는사람 휴대전화
- 구매한 이용권의 대한 잔여권수를 선물할 수 있습니다.
- 열람권은 1인당 1권씩 선물 가능합니다.
- 선물한 열람권이 ‘미등록’ 상태일 경우에만 ‘열람권 선물내역’화면에서 선물취소 가능합니다.
- 선물한 열람권의 등록유효기간은 14일 입니다.
(상대방이 기한내에 등록하지 않을 경우 소멸됩니다.) - 무제한 이용권일 경우 열람권 선물이 불가합니다.
첫 구매 시 교보e캐시 지급해 드립니다.
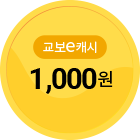
- 첫 구매 후 3일 이내 다운로드 시 익일 자동 지급
- 한 ID당 최초 1회 지급 / sam 이용권 제외
- 구글바이액션을 통해 교보eBook 구매 이력이 없는 회원 대상
- 교보e캐시 1,000원 지급 (유효기간 지급일로부터 7일)