Clean Code in C#
2020년 07월 17일 출간
- eBook 상품 정보
- 파일 정보 pdf (7.71MB)
- ISBN 9781838985691
- 쪽수 487쪽
- 지원기기 교보eBook App, PC e서재, 리더기, 웹뷰어
-
교보eBook App
듣기(TTS) 불가능
TTS 란?텍스트를 음성으로 읽어주는 기술입니다.
- 전자책의 편집 상태에 따라 본문의 흐름과 다르게 텍스트를 읽을 수 있습니다.
- 전자책 화면에 표기된 주석 등을 모두 읽어 줍니다.
- 이미지 형태로 제작된 전자책 (예 : ZIP 파일)은 TTS 기능을 지원하지 않습니다.
- '교보 ebook' 앱을 최신 버전으로 설치해야 이용 가능합니다. (Android v3. 0.26, iOS v3.0.09,PC v1.2 버전 이상)
PDF 필기 Android 가능 (iOS예정)
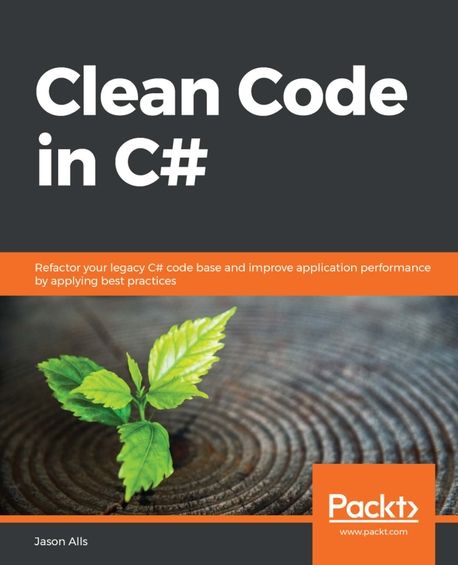
쿠폰적용가 24,300원
10% 할인 | 5%P 적립이 상품은 배송되지 않는 디지털 상품이며,
교보eBook앱이나 웹뷰어에서 바로 이용가능합니다.
카드&결제 혜택
- 5만원 이상 구매 시 추가 2,000P
- 3만원 이상 구매 시, 등급별 2~4% 추가 최대 416P
- 리뷰 작성 시, e교환권 추가 최대 300원
작품소개
이 상품이 속한 분야
Traditionally associated with developing Windows desktop applications and games, C# is now used in a wide variety of domains, such as web and cloud apps, and has become increasingly popular for mobile development. Despite its extensive coding features, professionals experience problems related to efficiency, scalability, and maintainability because of bad code. Clean Code in C# will help you identify these problems and solve them using coding best practices.
The book starts with a comparison of good and bad code, helping you understand the importance of coding standards, principles, and methodologies. You’ll then get to grips with code reviews and their role in improving your code while ensuring that you adhere to industry-recognized coding standards. This C# book covers unit testing, delves into test-driven development, and addresses cross-cutting concerns. You’ll explore good programming practices for objects, data structures, exception handling, and other aspects of writing C# computer programs. Once you’ve studied API design and discovered tools for improving code quality, you’ll look at examples of bad code and understand which coding practices you should avoid.
By the end of this clean code book, you’ll have the developed skills you need in order to apply industry-approved coding practices to write clean, readable, extendable, and maintainable C# code.
▶What You Will Learn
- Write code that allows software to be modified and adapted over time
- Implement the fail-pass-refactor methodology using a sample C# console application
- Address cross-cutting concerns with the help of software design patterns
- Write custom C# exceptions that provide meaningful information
- Identify poor quality C# code that needs to be refactored
- Secure APIs with API keys and protect data using Azure Key Vault
- Improve your code’s performance by using tools for profiling and refactoring
▶Key Features
- Write code that cleanly integrates with other systems while maintaining well-defined software boundaries
- Understand how coding principles and standards enhance software quality
- Learn how to avoid common errors while implementing concurrency or threading
▶Who This Book Is For
This coding book is for C# developers, team leads, senior software engineers, and software architects who want to improve the efficiency of their legacy systems. A strong understanding of C# programming is required.
1. Coding Standards and Principles in C#
2. Code Review ? Process and Importance
3. Classes, Objects, and Data Structures
4. Writing Clean Functions
5. Exception Handling
6. Unit Testing
7. End-to-End System Testing
8. Threading and Concurrency
9. Designing and Developing APIs
10. Securing APIs with API Keys and Azure Key Vault
11. Addressing Cross-Cutting Concerns
12. Using Tools to Improve Code Quality
13. Refactoring C# Code ? Identifying Code Smells
14. Refactoring C# Code ? Implementing Design Patterns
▶What this book covers
- Chapter 1, Coding Standards and Principles in C#, looks at some good code contrasted with bad code. As you read through this chapter, you will come to understand why you need coding standards, principles, methodologies, and code conventions. You will learn about modularity and the design guidelines KISS, YAGNI, DRY, SOLID, and Occam's razor.
- Chapter 2, Code Review ? Process and Importance, takes you through the code review process and provides reasons for its importance. In this chapter, you are guided through the process of preparing code for review, leading a code review, knowing what to review, knowing when to send code for review, and how to provide and respond to review feedback.
- Chapter 3, Classes, Objects, and Data Structures, covers the broad topics of class organization, documentation comments, cohesion, coupling, the Law of Demeter, and immutable objects and data structures. By the end of the chapter, you will be able to write code that is well organized and only has a single responsibility, provide users of the code with relevant documentation, and make code extensible.
- Chapter 4, Writing Clean Functions, helps you to understand functional programming, how to keep methods small, and how to avoid code duplication and multiple parameters. By the time you finish this chapter, you will be able to describe functional programming, write functional code, avoid writing code with more than two parameters, write immutable data objects and structures, keep your methods small, and write code that adheres to the Single Responsibility Principle.
- Chapter 5, Exception Handling, covers checked and unchecked exceptions, NullPointerException, and how to avoid them as well as covering, business rule exceptions, providing meaningful data, and building your own custom exceptions.
- Chapter 6, Unit Testing, takes you through using the Behavior-Driven Development (BDD) software methodology using SpecFlow, and Test-Driven Development (TDD) using MSTest and NUnit. You will learn how to write mock (fake) objects using Moq, and how to use the TDD software methodology to write tests that fail, make the tests pass, and then refactor the code once it passes.
- Chapter 7, End-to-End System Testing, guides you through the manual process of end-toend testing using an example project. In this chapter, you will perform End-to-End (E2E) testing, code and test factories, code and test dependency injection, and test modularization. You will also learn how to utilize modularization.
- Chapter 8, Threading and Concurrency, focuses on understanding the thread life cycle; adding parameters to threads; using ThreadPool, mutexes, and synchronous threads; working with parallel threads using semaphores; limiting the number of threads and processors used by ThreadPool; preventing deadlocks and race conditions; static methods and constructors; mutability and immutability; and thread-safety.
- Chapter 9, Designing and Developing APIs, helps you to understand what an API is, API proxies, API design guidelines, API design using RAML, and Swagger API development. In this chapter, you will design a language-agnostic API in RAML and develop it in C#, and you will document your API using Swagger.
- Chapter 10, Securing APIs with API Keys and Azure Key Vault, shows you how to obtain a third-party API key, store that key in Azure Key Vault, and retrieve it via an API that you will build and deploy to Azure. You will then implement API key authentication and authorization to secure your own API.
- Chapter 11, Addressing Cross-Cutting Concerns, introduces you to using PostSharp to address cross-cutting concerns using aspects and attributes that form the basis of aspectoriented development. You will also learn how to use proxies and decorators.
- Chapter 12, Using Tools to Improve Code Quality, exposes you to various tools that will assist you in writing quality code and ...
▶ Preface
Welcome to Clean Code in C#. You will learn how to identify problematic code that, while it compiles, does not lend itself to readability, maintainability, and extensibility. You will also learn about various tools and patterns, along with ways to refactor code to make it clean.
작가정보
저자(글) Jason Alls
Jason Alls has been programming for over 21 years using Microsoft technologies. Working with an Australasian company, he started his career developing call center management reporting software used by global clients including telecom providers, banks, airlines, and the police. He then moved on to develop GIS marketing applications and worked in the banking sector performing data migrations between Oracle and SQL Server. Certified as an MCAD in C# since 2005, he has been involved in the development of various desktop, web, and mobile applications.Currently employed by a globally recognized leader in the educational software sector, he develops and supports dyslexia testing and assessment software written in ASP.NET, Angular, and C#.
이 상품의 총서
Klover리뷰 (0)
- - e교환권은 적립일로부터 180일 동안 사용 가능합니다.
- - 리워드는 1,000원 이상 eBook, 오디오북, 동영상에 한해 다운로드 완료 후 리뷰 작성 시 익일 제공됩니다.
- - 리워드는 한 상품에 최초 1회만 제공됩니다.
- - sam 이용권 구매 상품 / 선물받은 eBook은 리워드 대상에서 제외됩니다.
- 도서나 타인에 대해 근거 없이 비방을 하거나 타인의 명예를 훼손할 수 있는 리뷰
- 도서와 무관한 내용의 리뷰
- 인신공격이나 욕설, 비속어, 혐오 발언이 개재된 리뷰
- 의성어나 의태어 등 내용의 의미가 없는 리뷰
구매 후 리뷰 작성 시, e교환권 100원 적립
문장수집
- 구매 후 90일 이내에 문장 수집 등록 시 e교환권 100원을 적립해 드립니다.
- e교환권은 적립일로부터 180일 동안 사용 가능합니다.
- 리워드는 1,000원 이상 eBook에 한해 다운로드 완료 후 문장수집 등록 시 제공됩니다.
- 리워드는 한 상품에 최초 1회만 제공됩니다.
- sam 이용권 구매 상품/오디오북·동영상 상품/주문취소/환불 시 리워드 대상에서 제외됩니다.
구매 후 문장수집 작성 시, e교환권 100원 적립
신규가입 혜택 지급이 완료 되었습니다.
바로 사용 가능한 교보e캐시 1,000원 (유효기간 7일)
지금 바로 교보eBook의 다양한 콘텐츠를 이용해 보세요!
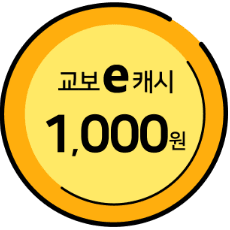
- 구매 후 90일 이내 작성 시, e교환권 100원 (최초1회)
- 리워드 제외 상품 : 마이 > 라이브러리 > Klover리뷰 > 리워드 안내 참고
- 콘텐츠 다운로드 또는 바로보기 완료 후 리뷰 작성 시 익일 제공
가장 와 닿는 하나의 키워드를 선택해주세요.
총 5MB 이하로 jpg,jpeg,png 파일만 업로드 가능합니다.
신고 사유를 선택해주세요.
신고 내용은 이용약관 및 정책에 의해 처리됩니다.
허위 신고일 경우, 신고자의 서비스 활동이 제한될 수
있으니 유의하시어 신중하게 신고해주세요.
이 글을 작성한 작성자의 모든 글은 블라인드 처리 됩니다.
구매 후 90일 이내 작성 시, e교환권 100원 적립
eBook 문장수집은 웹에서 직접 타이핑 가능하나, 모바일 앱에서 도서를 열람하여 문장을 드래그하시면 직접 타이핑 하실 필요 없이 보다 편하게 남길 수 있습니다.
차감하실 sam이용권을 선택하세요.
차감하실 sam이용권을 선택하세요.
선물하실 sam이용권을 선택하세요.
-
보유 권수 / 선물할 권수0권 / 1권
-
받는사람 이름받는사람 휴대전화
- 구매한 이용권의 대한 잔여권수를 선물할 수 있습니다.
- 열람권은 1인당 1권씩 선물 가능합니다.
- 선물한 열람권이 ‘미등록’ 상태일 경우에만 ‘열람권 선물내역’화면에서 선물취소 가능합니다.
- 선물한 열람권의 등록유효기간은 14일 입니다.
(상대방이 기한내에 등록하지 않을 경우 소멸됩니다.) - 무제한 이용권일 경우 열람권 선물이 불가합니다.
첫 구매 시 교보e캐시 지급해 드립니다.
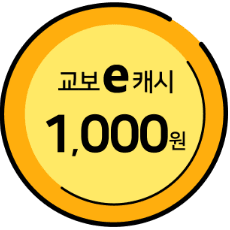
- 첫 구매 후 3일 이내 다운로드 시 익일 자동 지급
- 한 ID당 최초 1회 지급 / sam 이용권 제외
- 구글북액션을 통해 교보eBook 구매 이력이 없는 회원 대상
- 교보e캐시 1,000원 지급 (유효기간 지급일로부터 7일)